Sometimes you need to display dropdown spinner item with complex item consists not only string text, but with icon beside it. For example a dropdown spinner like the picture below showing a country list and its flag beside it.
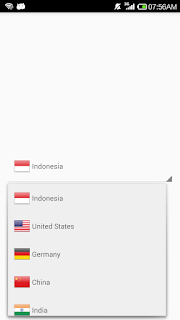 |
Custom spinner showing text and image |
Just follow the steps below to make that custom spinner.
1. Make activity layout file activity_spinner.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="12dp">
<Spinner
android:id="@+id/spinner"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_centerInParent="true">
</Spinner>
</RelativeLayout>
2. Make item layout file item_country.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal" android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center_vertical"
android:padding="12dp">
<ImageView
android:id="@+id/imgFlag"
android:layout_width="32dp"
android:layout_height="32dp" />
<TextView
android:id="@+id/textCountry"
android:layout_marginLeft="4dp"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"/>
</LinearLayout>
3. Make country class Country.java
package com.example.myapplication.spinner;
/**
* Created by SONY on 16/10/2016.
*/
public class Country {
public String name;
public int flag;
}
4. Make custom spinner adapter class CustomSpinnerAdapter.java
package com.example.myapplication.spinner;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.example.myapplication.R;
import java.util.List;
/**
* Created by SONY on 16/10/2016.
*/
public class CustomSpinnerAdapter extends ArrayAdapter<Country> {
private List<Country> data;
public CustomSpinnerAdapter(Context context, List<Country> data) {
super(context, 0, data);
this.data = data;
}
@Override
public View getDropDownView(int position, View convertView,ViewGroup parent) {
return getView(position, convertView, parent);
}
@Override
public View getView(int position, View convertView, ViewGroup parent){
Country country = data.get(position);
if(convertView == null) {
convertView = LayoutInflater.from(getContext()).inflate(R.layout.item_country, parent, false);
convertView.setTag(ViewHolder.createViewHolder(convertView));
}
ViewHolder holder = (ViewHolder)convertView.getTag();
holder.textCountry.setText(country.name);
holder.imgFlag.setImageResource(country.flag);
return convertView;
}
@Override
public int getCount( ) {
return data.size();
}
private static class ViewHolder {
public ImageView imgFlag;
public TextView textCountry;
public static ViewHolder createViewHolder(View view) {
ViewHolder holder = new ViewHolder();
holder.imgFlag = (ImageView) view.findViewById(R.id.imgFlag);
holder.textCountry = (TextView)view.findViewById(R.id.textCountry);
return holder;
}
}
}
5. Paste these png images into drawable folder
 |
flag_china.png |
 |
flag_germany.png |
 |
flag_india.png |
 |
flag_indonesia.png |
 |
flag_usa.png |
6. Make activity class SpinnerActivity.java
package com.example.myapplication.spinner;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.Spinner;
import com.example.myapplication.R;
import java.util.ArrayList;
import java.util.List;
/**
* Created by SONY on 16/10/2016.
*/
public class SpinnerActivity extends Activity {
private Spinner spinner;
private CustomSpinnerAdapter spinnerAdapter;
private List<Country> countries = new ArrayList<Country>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_spinner);
spinner = (Spinner) findViewById(R.id.spinner);
spinnerAdapter = new CustomSpinnerAdapter(this, countries );
spinner.setAdapter(spinnerAdapter);
spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
// do something after selected item here
Country country = countries.get(position);
}
@Override
public void onNothingSelected(AdapterView<?> parent) {
}
});
populateCountries();
}
private void populateCountries() {
Country indonesia = new Country();
indonesia.name = "Indonesia";
indonesia.flag = R.drawable.flag_indonesia;
Country usa = new Country();
usa.name = "United States";
usa.flag = R.drawable.flag_usa;
Country germany = new Country();
germany.name = "Germany";
germany.flag = R.drawable.flag_germany;
Country china = new Country();
china.name = "China";
china.flag = R.drawable.flag_china;
Country india = new Country();
india.name = "India";
india.flag = R.drawable.flag_india;
countries.add(indonesia);
countries.add(usa);
countries.add(germany);
countries.add(china);
countries.add(india);
spinnerAdapter.notifyDataSetChanged();
}
}
7. Make AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapplication">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name="com.example.myapplication.spinner.SpinnerActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
8. Now run the app. You should see a spinner showing country list with name and flag like in the
picture above.
Thank you for visiting our website. Just comment below if you have any question to ask.
No comments:
Post a Comment